The goal of this assignment is to take the digitized Prokudin-Gorskii
glass plate images and, using image processing techniques,
automatically produce a color image with as few visual artifacts as
possible. This process includes extracting the three color channel
images, placing them on top of each other, and aligning them so that
they form a single RGB color image.
Another goal of this project is to implement both a single-scale and a
multi-scale image alignment algorithm to account for smaller .jpg and
larger .tif files. On the right I have included a workflow image taken
from the CS 180 lecture 1 slide deck. I believe that it depicts a nice
overview of the project.
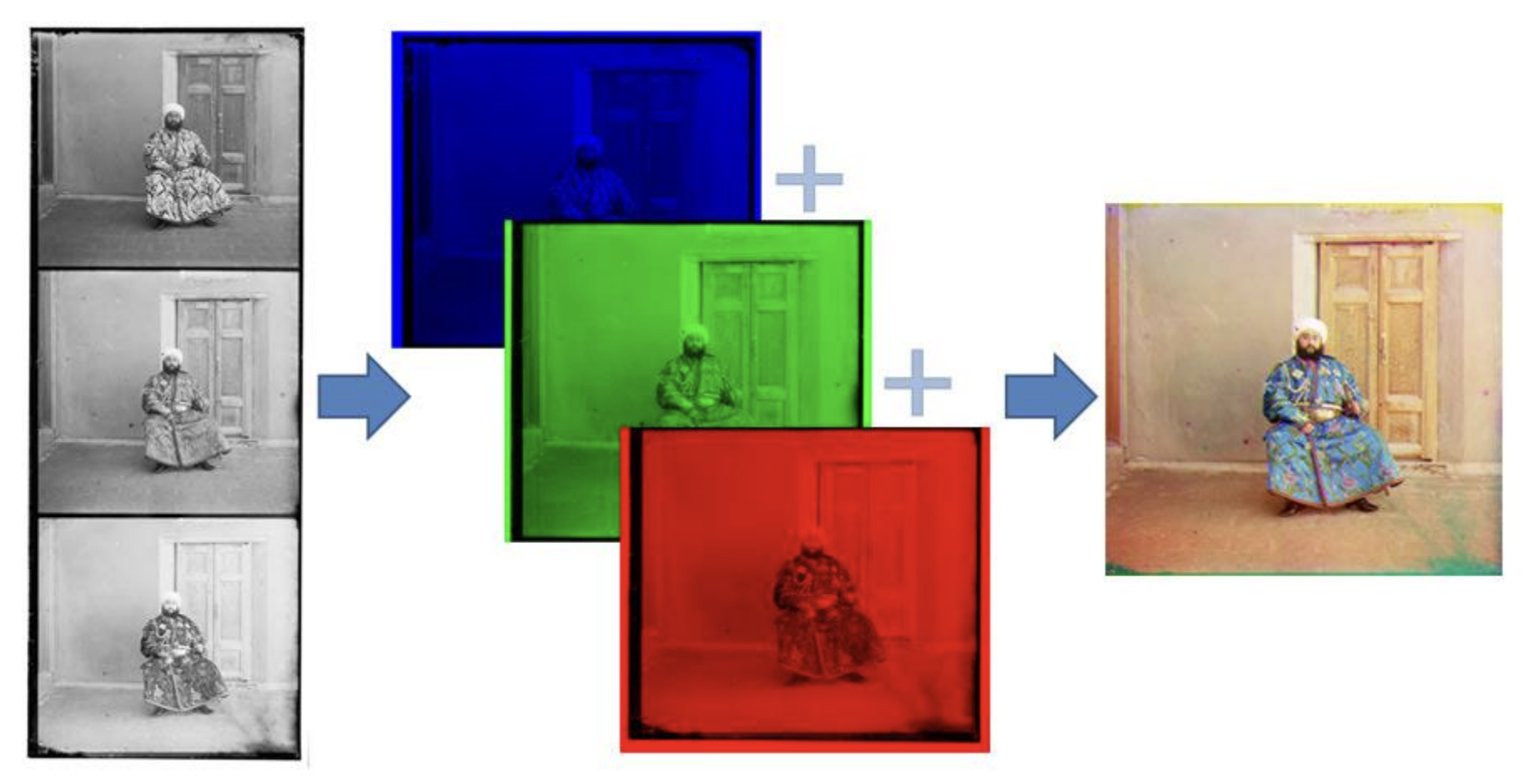